(I had done this two years ago and posted on my personal website. I never thought someone still sent email to me for this, even ask for source code. I think maybe more people want to know it. Posting on blogger is absolutely much popular than on my personal website).

It is built base on properJavaRDP, which is an open source Java RDP client for Windows Terminal Services. The idea of "seamless" is come from rdesktop, which is a open source RDP client on unix platform. "seamless" can make remote running application looks like running locally. I upgrad properJavaRDP code from AWT to Swing, and add the functionality to handle "seamless" message in a special rdp vchannel.
new update(2010.06): support window 2008 now (solve problem of “wrong modulus size! expected64+8got:264”)
new update(2010.06.17): support seamless mode applet
Seamless Java RDP client
It is a general Java RDP client for Windows Terminal Services with "seamless" feature. It is built base on properJavaRDP.
Product details
To use seamless feature, you need seamlessRDP server extension. A SeamlessRDP server extension is available to support integration of individual applications with the client desktop. Precompiled binaries are available from Cendio (If you want Connetion sharing feature, you have to get patch from http://www.fontis.com.au/rdesktop). My contribution is updated properJavaRDP code from AWT to Swing, and add the functionality to handle "seamless" message in a special rdp vchannel. jdk1.6.0-10 is needed, since shaping jframe is a new feature of jdk1.6.0-10.
To download the client jar file: javaRDP16-1.1.jar. A usage document is here:HowTo.
I also write a Adito extension: adito-application-seamlessjavardp.zip. Just for you convenience, i upload service side exe package here:seamlessrdp_server.zip.
(05/12/2009) Recently, i got chance to take a look at "rdesktop session connection-sharing" from http://www.fontis.com.au/rdesktop. It is a very interesting feature which can save TS session. I think it is good to port this feature to my seamless JavaRDPClient. It took me one day to do this. I have updated all above link with the new update. Welcome you to try this cool feature.
"Connection sharing - allows a single rdesktop connection to launch multiple applications. When run in seamless mode, rdesktop creates and listens on a control socket. A new option allows rdesktop to be run in slave mode, which notifies the master rdesktop instance of a new command to be run and then exits. The master instance sends a client-to-server message to the SeamlessRDP server component, which runs the new command".
see the difference of "seamless rdp" from normal rdp client with following screenshot:
1. nornal properJavaRdp client: ie and notepad is running on a remote server. you can see there is always a "frame" around the remote application.(My OS is english version XP, remote server is a chinese version windows 2003)
2. Seamless rdp client: ie and notepad is running on a remote server. you can are running like "local" application. (My OS is english version XP, remote server is a chinese version windows 2003)

The usage:
First, On windows as server
download seamlessrdp_server.zip and upzip it to C:\seamlessrdp
Then, on client side (windows or unix support jdk1.6u10),
Run the pure seamless client:
c:\>java -jar JavaRDP16-1.1.jar -u administrator -p 111111 -fs -s "C:\seamlessrdp\seamlessrdpshell.exe C:\Program Files\Internet Explorer\iexplore.exe" 192.168.1.135:3389
Run as seamless client with Connection-sharing feature:
1. start the firt client :
c:\>java -jar JavaRDP16-1.1.jar -u administrator -p 111111 -fm -s "C:\seamlessrdp\seamlessrdpshell.exe C:\Program Files\Internet Explorer\iexplore.exe" 192.168.1.135:3389
2. start more client but share the same connection with above one:
c:\>java -jar JavaRDP16-1.1.jar -fc -s "C:\Program Files\Internet Explorer\iexplore.exe"
------------------------------------------------------------------------------------------------------------------------
How to run as applet:
(take a look at exampleHtml to be aware of how to fill the params.)
First, the JavaRDP16-1.1.jar should be signed, and make sure it is in the right directory. I wrote a html(javaRdpApplet.html) and “sign.bat” in case you do not know how to sign and run the RDP jar.
Step by step:
1. create self certification and sign the jar package. In “sign.bat”, there are four commands to be executed:
keytool -genkey -dname "cn=BeanSoft Studio, ou=Java Software, o=BeanSoft Studio, c=China" -alias beansoft -keypass beansoft -storepass beansoft -validity 365 -keystore .\beansoft
keytool -list -keystore .\beansoft -storepass beansoft
keytool -export -keystore .\beansoft -storepass beansoft -file beansoft.cer -alias beansoft
jarsigner -verbose -keystore .\beansoft javaRDP16-1.1.jar beansoft
If you already have your certification file, you may just need to run the command in last line.
Before running sign.bat, the file list is as below:

2. run the sign.bat, you will get a window as below, input your password, in my case, it is “beansoft”.
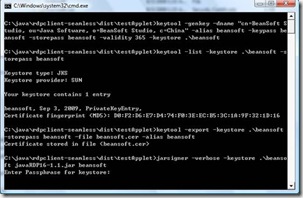
3. Now, you got file list should like:
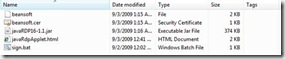
Notice that the size of javaRDP16-1.1.jar is bigger than original one. (in my testing, it is from 352KB to 374KB). It is becase it is signed. “jarsigner” add some file to META-INF.
4. Now you can try javaRdpApplet.html with IE explorer. I got message like below and it work well if you input correct IP address.
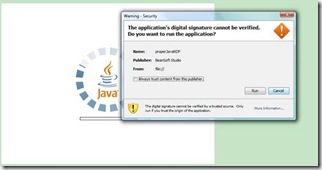